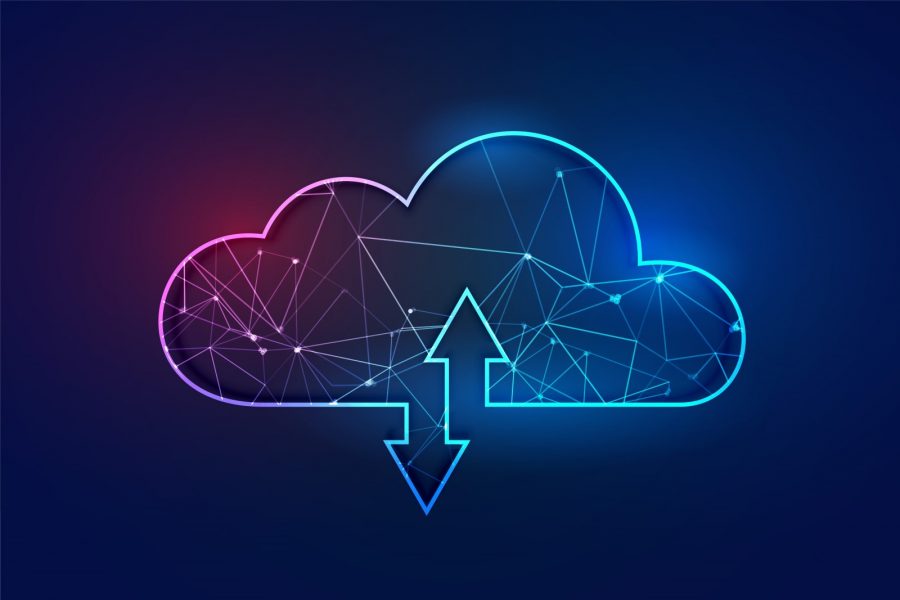
External Logging Feature in 01Cloud
An External Logging feature is a feature that is used to collect and store log data from various sources, such as servers, applications, and devices. Logging are typically designed to be deployed in a container orchestration platform such as Kubernetes and to work in concert with other tools such as log shippers, log aggregators, and…