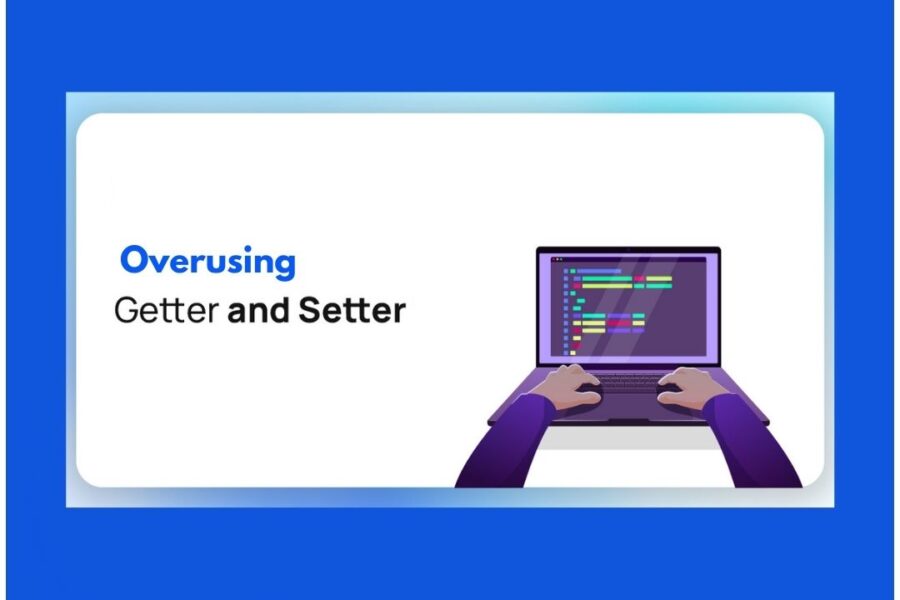
Overusing getters and setters
Encapsulation is used to hide the values or state of a structured data object, preventing unauthorized parties’ direct access to them. In Golang there is no by default support of getters and setters, so it is optional. There are few advantage of using getters and setters event in golang and they are mentaion below :-…
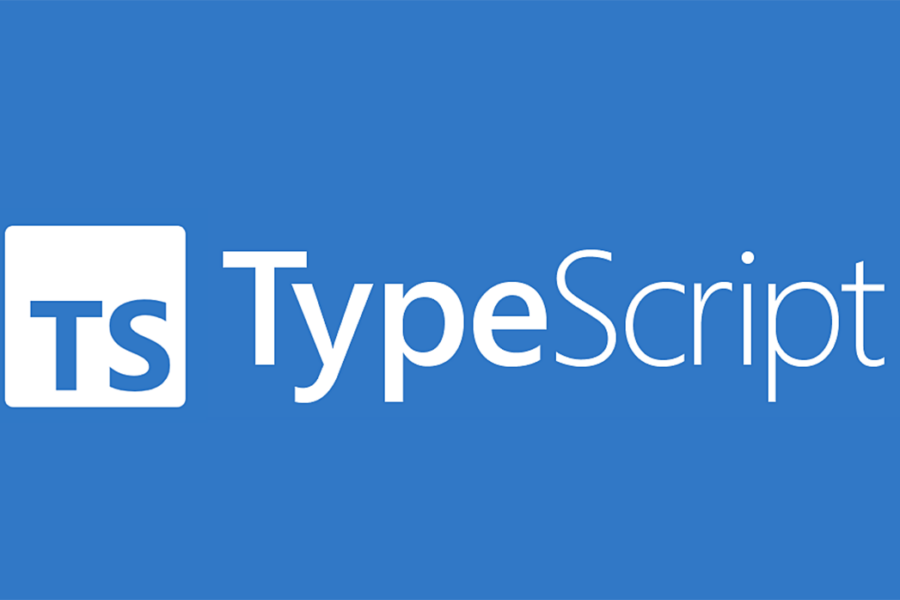
Avoid any Type in TS (anti-pattern)
What are types in TS? Types in TS helps us understand what methods & properties are associated with a given value/variable in a program that can help us analyze our code for existing errors and prevent further errors. For example a value that is assigned a type of a string tells us that the value…
Variable Shadowing in Go
As a part of this blog post, I will try to explain about variable shadowing and how to avoid it. In programming, scope of variable defines to the places a variable can be referenced. In Golang, a variable name declared in a block can be redeclared in an inner block. This mechanism is called variable shadowing….
Interface on producer side
Interfaces are used to create common abstractions that multiple objects can implement. Before delving into this topic, let’s make sure the terms we use throughout this section are clear: It’s common to see developers creating interfaces on the producer side, alongside the concrete implementation. But in Go, in most cases, this is not what we…
Unnecessary nested code
Readable code requires less cognitive effort to maintain a mental model; hence, it is easier to read and maintain. A critical aspect of readability is the number of nested levels. Code is qualified as readable based on multiple criteria such as naming, consistency, formatting, and so forth. While programming, we need to maintain mental models…
Being confused about when to use generics
Introduction The Go 1.18 release introduced a new feature called generic types (commonly known by the shorter term, generics). This allows writing code with types that can be specified later and instantiated when needed. However, it can be confusing about when to use generics and when not to. In this blog, I will try to describe the concept…
Optional Function Parameter Pattern
Go doesn’t support optional function parameters. However, the need for optional parameters will always exist. There are many ways to provide optional parameters to a function in Go, but the most graceful way is to use functional options. Do in this blog we will go through a concrete example and covers different ways to handle…
Project misorganization
Project organization is one of the most common mistake made by Go Developer. Go provides a lots of freedom for designing the packages and modules hence it is not easy task to organize the project. is The purpose of organizing the project are maintainability, readability, consistency and so on.
Any says nothing
The interface type that specifies zero methods is known as the empty interface: interface{} An empty interface may hold values of any type. (Every type implements at least zero methods.) Empty interfaces are used by code that handles values of unknown type. With Go 1.18 the predeclared type any became an alias for an empty…